240. Search a 2D Matrix II
1. Question
Write an efficient algorithm that searches for a target
value in an m x n
integer matrix. The matrix
has the following properties:
- Integers in each row are sorted in ascending from left to right.
- Integers in each column are sorted in ascending from top to bottom.
2. Examples
Example 1:
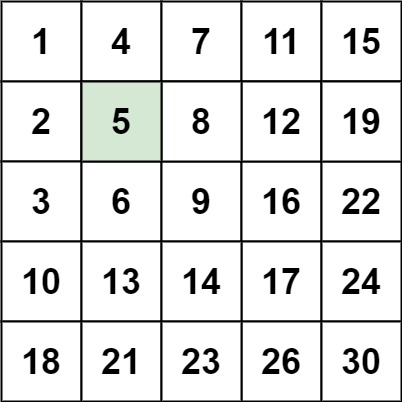
Input: matrix = [[1,4,7,11,15],[2,5,8,12,19],[3,6,9,16,22],[10,13,14,17,24],[18,21,23,26,30]], target = 5
Output: true
Example 2:
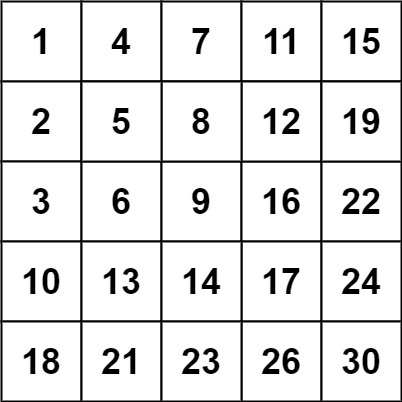
Input: matrix = [[1,4,7,11,15],[2,5,8,12,19],[3,6,9,16,22],[10,13,14,17,24],[18,21,23,26,30]], target = 20
Output: false
3. Constraints
m == matrix.length
n == matrix[i].length
1 <= n, m <= 300
- -109 <=
matrix[i][j]
<= 109 - All the integers in each row are sorted in ascending order.
- All the integers in each column are sorted in ascending order.
- -109 <= target <= 109
4. References
来源:力扣(LeetCode) 链接:https://leetcode-cn.com/problems/search-a-2d-matrix-ii 著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
5. Solutions
5.1. 暴力枚举
class Solution {
public boolean searchMatrix(int[][] matrix, int target) {
for (int i = 0; i < matrix.length; i++) {
for (int j = 0; j < matrix[0].length; j++) {
if (matrix[i][j] == target) {
return true;
}
}
}
return false;
}
}
5.2. 二分的变种
class Solution {
public boolean searchMatrix(int[][] matrix, int target) {
int m = matrix.length;
int n = matrix[0].length;
int x = 0;
int y = m - 1;
while (x < n && y >= 0) {
if (matrix[y][x] == target) {
return true;
} else if (matrix[y][x] < target) {
x++;
} else {
y--;
}
}
return false;
}
}